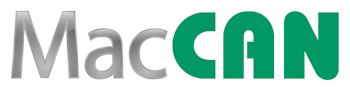 |
libPCBUSB
Version 0.13, Build 1978 of July 28, 2024
macOS Library for PCAN-USB Interfaces
|
Go to the documentation of this file.
49 #ifndef PCAN_API_H_INCLUDED
50 #define PCAN_API_H_INCLUDED
83 #define PCAN_NONEBUS 0x00U
85 #define PCAN_USBBUS1 0x51U
86 #define PCAN_USBBUS2 0x52U
87 #define PCAN_USBBUS3 0x53U
88 #define PCAN_USBBUS4 0x54U
89 #define PCAN_USBBUS5 0x55U
90 #define PCAN_USBBUS6 0x56U
91 #define PCAN_USBBUS7 0x57U
92 #define PCAN_USBBUS8 0x58U
94 #define PCAN_USBBUS9 0x509U
95 #define PCAN_USBBUS10 0x50AU
96 #define PCAN_USBBUS11 0x50BU
97 #define PCAN_USBBUS12 0x50CU
98 #define PCAN_USBBUS13 0x50DU
99 #define PCAN_USBBUS14 0x50EU
100 #define PCAN_USBBUS15 0x50FU
101 #define PCAN_USBBUS16 0x510U
107 #define PCAN_ERROR_OK 0x00000U
108 #define PCAN_ERROR_XMTFULL 0x00001U
109 #define PCAN_ERROR_OVERRUN 0x00002U
110 #define PCAN_ERROR_BUSLIGHT 0x00004U
111 #define PCAN_ERROR_BUSHEAVY 0x00008U
112 #define PCAN_ERROR_BUSWARNING PCAN_ERROR_BUSHEAVY
113 #define PCAN_ERROR_BUSPASSIVE 0x40000U
114 #define PCAN_ERROR_BUSOFF 0x00010U
115 #define PCAN_ERROR_ANYBUSERR (PCAN_ERROR_BUSWARNING | PCAN_ERROR_BUSLIGHT | PCAN_ERROR_BUSHEAVY | PCAN_ERROR_BUSOFF | PCAN_ERROR_BUSPASSIVE)
116 #define PCAN_ERROR_QRCVEMPTY 0x00020U
117 #define PCAN_ERROR_QOVERRUN 0x00040U
118 #define PCAN_ERROR_QXMTFULL 0x00080U
119 #define PCAN_ERROR_REGTEST 0x00100U
120 #define PCAN_ERROR_NODRIVER 0x00200U
121 #define PCAN_ERROR_HWINUSE 0x00400U
122 #define PCAN_ERROR_NETINUSE 0x00800U
123 #define PCAN_ERROR_ILLHW 0x01400U
124 #define PCAN_ERROR_ILLNET 0x01800U
125 #define PCAN_ERROR_ILLCLIENT 0x01C00U
126 #define PCAN_ERROR_ILLHANDLE (PCAN_ERROR_ILLHW | PCAN_ERROR_ILLNET | PCAN_ERROR_ILLCLIENT)
127 #define PCAN_ERROR_RESOURCE 0x02000U
128 #define PCAN_ERROR_ILLPARAMTYPE 0x04000U
129 #define PCAN_ERROR_ILLPARAMVAL 0x08000U
130 #define PCAN_ERROR_UNKNOWN 0x10000U
131 #define PCAN_ERROR_ILLDATA 0x20000U
132 #define PCAN_ERROR_ILLMODE 0x80000U
133 #define PCAN_ERROR_CAUTION 0x2000000U
134 #define PCAN_ERROR_INITIALIZE 0x4000000U
135 #define PCAN_ERROR_ILLOPERATION 0x8000000U
139 #define PCAN_NONE 0x00U
140 #define PCAN_PEAKCAN 0x01U
141 #define PCAN_ISA 0x02U
142 #define PCAN_DNG 0x03U
143 #define PCAN_PCI 0x04U
144 #define PCAN_USB 0x05U
145 #define PCAN_PCC 0x06U
146 #define PCAN_VIRTUAL 0x07U
147 #define PCAN_LAN 0x08U
151 #define PCAN_DEVICE_ID 0x01U
152 #define PCAN_5VOLTS_POWER 0x02U
153 #define PCAN_RECEIVE_EVENT 0x03U
154 #define PCAN_MESSAGE_FILTER 0x04U
155 #define PCAN_API_VERSION 0x05U
156 #define PCAN_CHANNEL_VERSION 0x06U
157 #define PCAN_BUSOFF_AUTORESET 0x07U
158 #define PCAN_LISTEN_ONLY 0x08U
159 #define PCAN_LOG_LOCATION 0x09U
160 #define PCAN_LOG_STATUS 0x0AU
161 #define PCAN_LOG_CONFIGURE 0x0BU
162 #define PCAN_LOG_TEXT 0x0CU
163 #define PCAN_CHANNEL_CONDITION 0x0DU
164 #define PCAN_HARDWARE_NAME 0x0EU
165 #define PCAN_RECEIVE_STATUS 0x0FU
166 #define PCAN_CONTROLLER_NUMBER 0x10U
167 #define PCAN_TRACE_LOCATION 0x11U
168 #define PCAN_TRACE_STATUS 0x12U
169 #define PCAN_TRACE_SIZE 0x13U
170 #define PCAN_TRACE_CONFIGURE 0x14U
171 #define PCAN_CHANNEL_IDENTIFYING 0x15U
172 #define PCAN_CHANNEL_FEATURES 0x16U
173 #define PCAN_BITRATE_ADAPTING 0x17U
174 #define PCAN_BITRATE_INFO 0x18U
175 #define PCAN_BITRATE_INFO_FD 0x19U
176 #define PCAN_BUSSPEED_NOMINAL 0x1AU
177 #define PCAN_BUSSPEED_DATA 0x1BU
178 #define PCAN_IP_ADDRESS 0x1CU
179 #define PCAN_LAN_SERVICE_STATUS 0x1DU
180 #define PCAN_ALLOW_STATUS_FRAMES 0x1EU
181 #define PCAN_ALLOW_RTR_FRAMES 0x1FU
182 #define PCAN_ALLOW_ERROR_FRAMES 0x20U
183 #define PCAN_INTERFRAME_DELAY 0x21U
184 #define PCAN_ACCEPTANCE_FILTER_11BIT 0x22U
185 #define PCAN_ACCEPTANCE_FILTER_29BIT 0x23U
186 #define PCAN_IO_DIGITAL_CONFIGURATION 0x24U
187 #define PCAN_IO_DIGITAL_VALUE 0x25U
188 #define PCAN_IO_DIGITAL_SET 0x26U
189 #define PCAN_IO_DIGITAL_CLEAR 0x27U
190 #define PCAN_IO_ANALOG_VALUE 0x28U
191 #define PCAN_FIRMWARE_VERSION 0x29U
192 #define PCAN_ATTACHED_CHANNELS_COUNT 0x2AU
193 #define PCAN_ATTACHED_CHANNELS 0x2BU
194 #define PCAN_ALLOW_ECHO_FRAMES 0x2CU
195 #define PCAN_DEVICE_PART_NUMBER 0x2DU
196 #define PCAN_HARD_RESET_STATUS 0x2EU
197 #define PCAN_LAN_CHANNEL_DIRECTION 0x2FU
198 #define PCAN_EXT_BTR0BTR1 0x80U
199 #define PCAN_EXT_TX_COUNTER 0x81U
200 #define PCAN_EXT_RX_COUNTER 0x82U
201 #define PCAN_EXT_ERR_COUNTER 0x83U
202 #define PCAN_EXT_RX_QUE_OVERRUN 0x84U
203 #define PCAN_EXT_HARDWARE_VERSION 0x85U
204 #define PCAN_EXT_SOFTWARE_VERSION 0x86U
205 #define PCAN_EXT_RECEIVE_CALLBACK 0x87U
206 #define PCAN_EXT_LOG_USB 0x8FU
210 #define PCAN_DEVICE_NUMBER PCAN_DEVICE_ID
214 #define PCAN_PARAMETER_OFF 0x00U
215 #define PCAN_PARAMETER_ON 0x01U
216 #define PCAN_FILTER_CLOSE 0x00U
217 #define PCAN_FILTER_OPEN 0x01U
218 #define PCAN_FILTER_CUSTOM 0x02U
219 #define PCAN_CHANNEL_UNAVAILABLE 0x00U
220 #define PCAN_CHANNEL_AVAILABLE 0x01U
221 #define PCAN_CHANNEL_OCCUPIED 0x02U
222 #define PCAN_CHANNEL_PCANVIEW (PCAN_CHANNEL_AVAILABLE | PCAN_CHANNEL_OCCUPIED)
224 #define LOG_FUNCTION_DEFAULT 0x00U
225 #define LOG_FUNCTION_ENTRY 0x01U
226 #define LOG_FUNCTION_PARAMETERS 0x02U
227 #define LOG_FUNCTION_LEAVE 0x04U
228 #define LOG_FUNCTION_WRITE 0x08U
229 #define LOG_FUNCTION_READ 0x10U
230 #define LOG_FUNCTION_ALL 0xFFFFU
232 #define TRACE_FILE_SINGLE 0x00U
233 #define TRACE_FILE_SEGMENTED 0x01U
234 #define TRACE_FILE_DATE 0x02U
235 #define TRACE_FILE_TIME 0x04U
236 #define TRACE_FILE_OVERWRITE 0x80U
237 #define TRACE_FILE_DATA_LENGTH 0x100U
239 #define FEATURE_FD_CAPABLE 0x01U
240 #define FEATURE_DELAY_CAPABLE 0x02U
241 #define FEATURE_IO_CAPABLE 0x04U
243 #define SERVICE_STATUS_STOPPED 0x01U
244 #define SERVICE_STATUS_RUNNING 0x04U
246 #define LAN_DIRECTION_READ 0x01U
247 #define LAN_DIRECTION_WRITE 0x02U
248 #define LAN_DIRECTION_READ_WRITE (LAN_DIRECTION_READ | LAN_DIRECTION_WRITE) // The PCAN-Channel communication is bidirectional
252 #define MAX_LENGTH_HARDWARE_NAME 33
253 #define MAX_LENGTH_VERSION_STRING 256
257 #define PCAN_MESSAGE_STANDARD 0x00U
258 #define PCAN_MESSAGE_RTR 0x01U
259 #define PCAN_MESSAGE_EXTENDED 0x02U
260 #define PCAN_MESSAGE_FD 0x04U
261 #define PCAN_MESSAGE_BRS 0x08U
262 #define PCAN_MESSAGE_ESI 0x10U
263 #define PCAN_MESSAGE_ECHO 0x20U
264 #define PCAN_MESSAGE_ERRFRAME 0x40U
265 #define PCAN_MESSAGE_STATUS 0x80U
269 #define LOOKUP_DEVICE_TYPE __T("devicetype")
270 #define LOOKUP_DEVICE_ID __T("deviceid")
271 #define LOOKUP_CONTROLLER_NUMBER __T("controllernumber")
272 #define LOOKUP_IP_ADDRESS __T("ipaddress")
276 #define PCAN_MODE_STANDARD PCAN_MESSAGE_STANDARD
277 #define PCAN_MODE_EXTENDED PCAN_MESSAGE_EXTENDED
284 #define PCAN_BAUD_1M 0x0014U
285 #define PCAN_BAUD_800K 0x0016U
286 #define PCAN_BAUD_500K 0x001CU
287 #define PCAN_BAUD_250K 0x011CU
288 #define PCAN_BAUD_125K 0x031CU
289 #define PCAN_BAUD_100K 0x432FU
290 #define PCAN_BAUD_95K 0xC34EU
291 #define PCAN_BAUD_83K 0x852BU
292 #define PCAN_BAUD_50K 0x472FU
293 #define PCAN_BAUD_47K 0x1414U
294 #define PCAN_BAUD_33K 0x8B2FU
295 #define PCAN_BAUD_20K 0x532FU
296 #define PCAN_BAUD_10K 0x672FU
297 #define PCAN_BAUD_5K 0x7F7FU
307 #define PCAN_BR_CLOCK __T("f_clock")
308 #define PCAN_BR_CLOCK_MHZ __T("f_clock_mhz")
309 #define PCAN_BR_NOM_BRP __T("nom_brp")
310 #define PCAN_BR_NOM_TSEG1 __T("nom_tseg1")
311 #define PCAN_BR_NOM_TSEG2 __T("nom_tseg2")
312 #define PCAN_BR_NOM_SJW __T("nom_sjw")
313 #define PCAN_BR_NOM_SAMPLE __T("nom_sam")
314 #define PCAN_BR_DATA_BRP __T("data_brp")
315 #define PCAN_BR_DATA_TSEG1 __T("data_tseg1")
316 #define PCAN_BR_DATA_TSEG2 __T("data_tseg2")
317 #define PCAN_BR_DATA_SJW __T("data_sjw")
318 #define PCAN_BR_DATA_SAMPLE __T("data_ssp_offset")
323 #define TPCANHandle WORD
324 #define TPCANStatus DWORD
325 #define TPCANParameter BYTE
326 #define TPCANDevice BYTE
327 #define TPCANMessageType BYTE
328 #define TPCANType BYTE
329 #define TPCANMode BYTE
330 #define TPCANBaudrate WORD
331 #define TPCANBitrateFD LPSTR
332 #define TPCANTimestampFD UINT64
TPCANMessageType MSGTYPE
Type of the message.
Definition: PCBUSB.h:359
TPCANStatus CAN_Write(TPCANHandle Channel, TPCANMsg *MessageBuffer)
Transmits a CAN message.
TPCANStatus CAN_FilterMessages(TPCANHandle Channel, DWORD FromID, DWORD ToID, TPCANMode Mode)
Configures the reception filter.
TPCANStatus CAN_GetErrorText(TPCANStatus Error, WORD Language, LPSTR Buffer)
Returns a descriptive text of a given TPCANStatus error code, in any desired language.
struct tagTPCANMsg TPCANMsg
TPCANStatus CAN_GetStatus(TPCANHandle Channel)
Gets the current status of a PCAN Channel.
#define _DEF_ARG
Definition: PCBUSB.h:389
TPCANStatus CAN_Reset(TPCANHandle Channel)
Resets the receive and transmit queues of the PCAN Channel.
TPCANStatus CAN_Read(TPCANHandle Channel, TPCANMsg *MessageBuffer, TPCANTimestamp *TimestampBuffer)
Reads a CAN message from the receive queue of a PCAN Channel.
struct tagTPCANChannelInformation TPCANChannelInformation
#define MAX_LENGTH_HARDWARE_NAME
Maximum length of the name of a device: 32 characters + terminator.
Definition: PCBUSB.h:252
struct tagTPCANTimestamp TPCANTimestamp
TPCANStatus CAN_SetValue(TPCANHandle Channel, TPCANParameter Parameter, void *Buffer, DWORD BufferLength)
Configures or sets a PCAN Channel value.
TPCANStatus CAN_WriteFD(TPCANHandle Channel, TPCANMsgFD *MessageBuffer)
Transmits a CAN message over a FD capable PCAN Channel.
#define TPCANDevice
Represents a PCAN device.
Definition: PCBUSB.h:326
#define TPCANStatus
Represents a PCAN status/error code (ATTENTION: changed from 64-bit to 32-bit)
Definition: PCBUSB.h:324
TPCANStatus CAN_GetValue(TPCANHandle Channel, TPCANParameter Parameter, void *Buffer, DWORD BufferLength)
Retrieves a PCAN Channel value.
WORD millis_overflow
Roll-arounds of millis.
Definition: PCBUSB.h:350
TPCANMessageType MSGTYPE
Type of the message.
Definition: PCBUSB.h:339
TPCANStatus CAN_InitializeFD(TPCANHandle Channel, TPCANBitrateFD BitrateFD)
Initializes a FD capable PCAN Channel.
TPCANStatus CAN_LookUpChannel(LPSTR Parameters, TPCANHandle *FoundChannel)
Finds a PCAN-Basic channel that matches with the given parameters.
#define TPCANType
Represents the type of PCAN hardware to be initialized.
Definition: PCBUSB.h:328
WORD micros
Microseconds: 0..999.
Definition: PCBUSB.h:351
#define TPCANMessageType
Represents the type of a PCAN message.
Definition: PCBUSB.h:327
#define TPCANTimestampFD
Represents a timestamp of a received PCAN FD message.
Definition: PCBUSB.h:332
TPCANStatus CAN_Initialize(TPCANHandle Channel, TPCANBaudrate Btr0Btr1, TPCANType HwType _DEF_ARG, DWORD IOPort _DEF_ARG, WORD Interrupt _DEF_ARG)
Initializes a PCAN Channel.
TPCANStatus CAN_Uninitialize(TPCANHandle Channel)
Uninitializes one or all PCAN Channels initialized by CAN_Initialize.
#define TPCANHandle
Represents a PCAN hardware channel handle.
Definition: PCBUSB.h:323
BYTE DATA[8]
Data of the message (DATA[0]..DATA[7])
Definition: PCBUSB.h:341
struct tagTPCANMsgFD TPCANMsgFD
TPCANStatus CAN_ReadFD(TPCANHandle Channel, TPCANMsgFD *MessageBuffer, TPCANTimestampFD *TimestampBuffer)
Reads a CAN message from the receive queue of a FD capable PCAN Channel.
DWORD millis
Base-value: milliseconds: 0.. 2^32-1.
Definition: PCBUSB.h:349
#define TPCANBitrateFD
Represents a PCAN-FD bit rate string.
Definition: PCBUSB.h:331
#define TPCANMode
Represents a PCAN filter mode.
Definition: PCBUSB.h:329
BYTE DATA[64]
Data of the message (DATA[0]..DATA[63])
Definition: PCBUSB.h:361
DWORD ID
11/29-bit message identifier
Definition: PCBUSB.h:358
#define TPCANBaudrate
Represents a PCAN Baud rate register value.
Definition: PCBUSB.h:330
BYTE DLC
Data Length Code of the message (0..15)
Definition: PCBUSB.h:360
DWORD ID
11/29-bit message identifier
Definition: PCBUSB.h:338
#define TPCANParameter
Represents a PCAN parameter to be read or set.
Definition: PCBUSB.h:325
BYTE LEN
Data Length Code of the message (0..8)
Definition: PCBUSB.h:340